A Little Order: Delving into the STL sorting algorithms
Speaker: Fred Tingaud
Audience level: Beginner | Intermediate
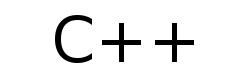
Benchmarking STL sorting algorithms can lead to surprises. For example, std::partial_sort takes considerably more time to sort half a vector than std::sort to sort it completely...
Starting from this counter-intuitive result, we'll engage on a journey where we'll look at the standard, read implementations of the STL and benchmark code to understand how std::sort, std::nth_element and std::partial_sort are implemented and why. In the process, we'll see some of the challenges STL implementers encountered and how they chose to overcome them.
Clean(er) Code for Large Scale Legacy Applications
Speaker: Arne Mertz
Audience level: Beginner | Intermediate | Advanced
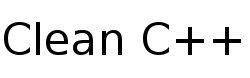
Due to the complexity of the language and the presence of some low-level language features, "Clean C++" seems to be an oxymoron for many developers. Especially in enterprise land, C++ applications tend to have large code bases grown over several years. Those legacy code bases tend to suffer from underdeveloped or missing unit and integration tests. Development teams maintaining such code bases resign over time and adopt "don't touch it, you'll break it" policies.
Nevertheless, it is possible to write clean C++, to at refactor even large C++ code bases as needed and cover critical parts with automated tests. The key elements to successfully regain control over code quality are a dedicated team and a set of properly sized and prioritized steps towards that goal.
Sane and safe C++ class types
Speaker: Peter Sommerlad
Audience level: Beginner | Intermediate
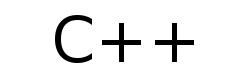
C++ is a complex language and with the introduction of move semantics, noexcept and constexpr in C++11 and later, defining or declaring the right combination of magic keywords in the right place is daunting for the uninitiated.
Engineering Software: Integral Types
Speaker: Andrei Zlate-Podani
Audience level: Intermediate | Advanced
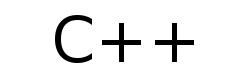
In spite of more than 40 years of programming practice, we still make even the most basic errors of API design and coding: integer overflow, error prone floating point arithmetic, unconstrained / flawed templated interfaces.
The Hitchhiker's Guide to Faster Builds
Speaker: Viktor Kirilov
Audience level: Intermediate | Advanced
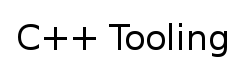
C++ is notorious for things such as performance, expressiveness, the lack of a standard build system and package management, complexity and long compile times.
The inability to iterate quickly is one of the biggest killers of productivity. This talk is aimed at anyone interested in improving the last of these points - it will provide insights into why compilation (and linking) take so long for C++ and will then provide an exhaustive list of techniques and tools to mitigate the problem, such as:
- tooling and infrastructure - hardware, build systems, caching, distributed builds, diagnostics of bottlenecks, code hygiene
- techniques - unity builds, precompiled headers, linking (static vs shared libraries)
- source code modification - the PIMPL idiom, better template use, annotations
- modules - what they are, when they are coming to C++ and what becomes obsolete because of them
Building a C++ Reflection System in One Weekend Using Clang and LLVM
Speaker: Arvid Gerstmann
Audience level: Intermediate | Advanced
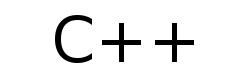
Designing and building the runtime reflection system from tomorrow, today! With the introduction of libraries like LLVM and libclang, building custom tools for C++ is more approachable than it has ever been before. With Clang we have a fantastic tool to analyze and augment the C++ AST without writing our own compiler or parser. In this talk we'll go over the design and implementation of a runtime reflection system, demonstrating the use of Clang and the LLVM framework to craft custom C++ tools for your own needs. We'll be able to query the members of a class, inspect their types and size, as well as qualifiers and user-definable flags. To show-case a real-world usage of the solution, we'll add a way to easily serialize any class to or from a byte stream, by making use of the reflection information. The implementation is available under a permissive open-source license on Github (https://github.com/leandros/metareflect).
...Better C++14 Reflections Without Macros, Markup nor External Tooling
Speaker: Antony Polukhin
Audience level: Intermediate | Advanced
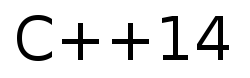
C++ was lacking the reflections feature for a long time. But a new metaprogramming trick was discovered recently: we can get some information about structure by probing it's braced initializes. Combining that trick with variadic templates, constexpr functions, implicit conversion operators, SFINAE, C++ core issues, decltype and integral constants we can count structure's fields and even deduce type of each field.
Now...
Best Practices for Concurrency
Speaker: Rainer Grimm
Audience level: Beginner | Intermediate
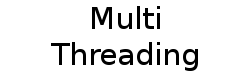
With the standardisation of C++11, C++ got a multithreading library and a memory model. The library has the basic building blocks such as atomics, threads, tasks, locks, and condition variables. The memory model provides guarantees for the thread-safe usage of this basic building blocks.
How to write more reliable code?
Speaker: Egor Bredikhin
Audience level: Beginner | Intermediate
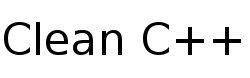
As a developer of a static analyzer, I have to review lots of defects in C++ code.
Many of them could have been avoided by using modern C++ techniques and additional code quality tools.
In this talk I'll go over a bunch of methods, improving code quality, such as:
unit testing, guidelines, modern C++ standard, code review, static analysis, dynamic analysis and even more.
We will consider some errors from real projects, and will discuss how we can prevent the same errors.
Modern approach to template meta-programming
Speaker: Ivan Čukić
Audience level: Intermediate
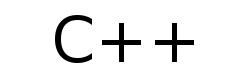
C++ has always had a powerful meta-programming sub-language which allowed library developers to perform magical feats like static introspection to achieve polymorhpic execution without inheritance. The problem was that the syntax was awkward and unnecessarily verbose which made learning meta-programming a daunting task.
Data-oriented design in practice
Speaker: Stoyan Nikolov
Audience level: Intermediate | Advanced
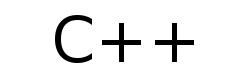
For decades C++ developers have built software around OOP concepts that ultimately didn’t deliver - we didn’t see the promises of code reuse, maintenance or simplicity fulfilled, and performance suffers significantly. Data-oriented design can be a better paradigm in fields where C++ is most important - game development, high-performance computing, and real-time systems.
Regular Types and Why Do I Care ?
Speaker: Victor Ciura
Audience level: Beginner | Intermediate
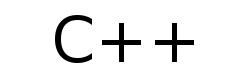
“Regular” is not exactly a new concept (pun intended). If we reflect back on STL and its design principles, as best described by Alexander Stepanov in his 1998 “Fundamentals of Generic Programming” paper or his lecture on this topic, from 2002, we see that regular types naturally appear as necessary foundational concepts in programming.
Taming Dynamic Memory - An Introduction to Custom Allocators
Speaker: Andreas Weis
Audience level: Beginner | Intermediate
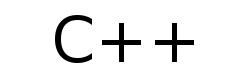
Dynamic memory allocation is a feature that is often taken for granted. Most developers use some form of new or malloc every day, usually without worrying too much what goes on behind the scenes. But what about those situations where the built-in mechanisms are not good enough, be it for reasons of performance, safety, or due to restrictions of the target hardware?
Cross-platform C++ development is challenging - let tools help!
Speaker: Marc Goodner
Audience level: Beginner | Intermediate
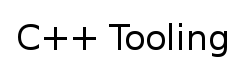
Writing high-quality error-free C++ code itself is a challenging task, let alone when having to juggle multiple platforms at the same time! In this session, we will talk about the many challenges in cross-platform C++ development and how tools can help: what options do I have if my production environment is different than my dev box? Can I be as productive when working with remote Linux machines? Is there any good C++ editor that works consistently on all platforms I work on? How can I efficiently build and debug CMake projects, and even get IntelliSense? How can I easily find and use open sourced libraries? Is there an easy way to write and run cross-platform tests? Come to this demo-heavy talk to see what Visual Studio 2017, Visual Studio Code, CMake, WSL, Vcpkg and more have to offer to make cross-platform C++ development much easier.
...Text Formatting For a Future Range-Based Standard Library
Speaker: Arno Schoedl
Audience level: Beginner | Intermediate | Advanced
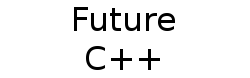
Text formatting has been a favorite problem of C++ library authors for a long time. The standard C++ iostreams have been criticized for being difficult to use due to their statefulness and slow due to runtime polymorphism. Despite its age, printf is still popular because of simplicity and speed. The Boost library offers two more alternatives, Boost.Format and Boost.LexicalCast. And finally, the P0645 standard proposal sponsored by Facebook is currently finding its way through the C++ committee.
All...